The Singleton Design Pattern: A Comprehensive Guide with Real-Life Applications
- sujosutech
- Nov 29, 2024
- 3 min read
Updated: Jan 24
Design patterns are essential tools in a developer’s arsenal, enabling the creation of scalable, maintainable, and efficient software. Among these, the Singleton Design Pattern stands out as one of the most widely used and straightforward patterns. In this article, we’ll explore the Singleton pattern in depth, understand its benefits, and see how it applies to real-world scenarios, making it a must-know for developers across industries.
What is the Singleton Design Pattern?
The Singleton design pattern ensures that only one instance of a class is created and provides a global point of access to that instance. This is particularly useful when one and only one object is needed to coordinate actions across the system.
Key Characteristics of Singleton
Single Instance: Ensures only one instance of the class exists.
Global Access Point: Provides a way to access the single instance globally.
Lazy Initialization: Optionally delays instance creation until it is first needed.
Why Use the Singleton Pattern?
Resource Management: Avoid multiple resource-consuming instances (e.g., database connections).
Consistency: Maintain a single source of truth for shared data or services.
Global Access: Enable easy access to the same instance from anywhere in the application.
How to Implement Singleton
Below is a platform-agnostic implementation, adaptable to most programming languages:
Pseudo-Code Implementation
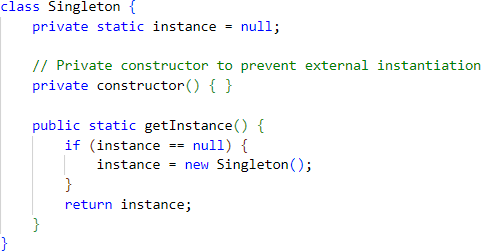
Real-World Applications of Singleton
1. Logging Systems
Why?: Logs need to be consistently stored in one location.
Example:
A logging service writes log entries to a file or console. Using multiple loggers might lead to redundant or conflicting entries.
2. Configuration Management
Why?: Centralized management of settings ensures consistency.
Example:
A web application fetching API keys, database URLs, or feature toggles uses a Singleton for uniform access.
3. Database Connection Pool
Why?: Establishing a new database connection is resource-intensive.
Example:
Applications like e-commerce platforms use a Singleton to share database connections and reduce overhead.
4. Thread Pool Management
Why?: Thread pools are costly to initialize and need centralized control.
Example:
Multi-threaded server applications use a Singleton thread pool to allocate tasks efficiently.
5. Caching
Why?: Avoid repeated calculations or database queries by storing frequently accessed data.
Example:
Applications with frequently accessed user profiles or product details use Singleton-based caches for speed.
Industry Applications
Banking and Finance:
Managing transaction logs and audit trails using a centralized logger.
E-Commerce:
Sharing shopping cart details across multiple sessions in an application.
IoT Systems:
Centralized management of devices and sensors using Singleton controllers.
Cloud Services:
Centralized resource allocation across multiple virtual machines.
Advantages of Singleton
Efficient Resource Usage: Reduces redundant initialization of heavy objects.
Controlled Access: Provides a single point of entry, enhancing security.
Global State Management: Simplifies sharing states across application modules.
Best Practices for Singleton
Thread Safety:
Ensure that the Singleton implementation works in a multi-threaded environment. Use double-checked locking for optimal performance.
2. Lazy Initialization:
Initialize the Singleton instance only when it's first accessed to save resources.
3. Dependency Injection:
Avoid hardcoding Singleton instances. Use dependency injection frameworks to manage lifecycle and testing.
4. Avoid Overuse:
Use Singletons sparingly to prevent tight coupling and global state abuse.
When Should You Use Singleton?
Singleton is Ideal for:
Shared Resources: Database connections, thread pools, or caches.
Global State: Logging services, configuration settings, or service registries.
Control Mechanisms: Centralized workflow managers or schedulers.
Avoid Singleton When:
You need multiple independent instances.
It leads to excessive coupling between modules.
Testing becomes challenging due to global state interference.
Real-Life Example: Singleton in Action
Global Logging Service
Imagine a large-scale web application where every module needs to log messages. Without a Singleton logger:
Each module creates its own logger.
Logs are scattered across multiple files or systems.
With Singleton:
A single logger instance writes to one centralized log file, ensuring consistency and reducing redundancy.
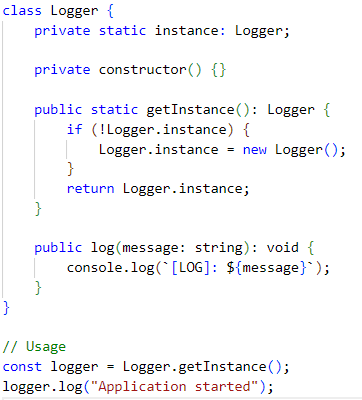
Conclusion
The Singleton Design Pattern is a powerful tool for ensuring controlled, efficient access to shared resources in software applications. From logging systems to database connections, it serves as a cornerstone of modern development across industries.
When used correctly, Singleton simplifies architecture and ensures scalability. However, like any tool, it should be applied thoughtfully to avoid unnecessary coupling or complexity. By following best practices and understanding its ideal use cases, you can leverage Singleton to build robust, high-performance systems.
コメント