Mastering SOLID Principles: The Blueprint for Scalable and Maintainable Software
- sujosutech
- Nov 29, 2024
- 3 min read
Updated: Jan 24
In the world of software development, building scalable, maintainable, and robust systems is critical. SOLID principles, a set of five core object-oriented design principles, provide the foundation for achieving these goals. Coined by Robert C. Martin (Uncle Bob), SOLID principles empower developers to write cleaner, more efficient code that is easy to understand, test, and modify.
In this article, we’ll explore each principle, its importance, and practical examples to help you master these concepts and apply them to real-world projects.
What Are the SOLID Principles?
The SOLID acronym represents five principles:
Single Responsibility Principle (SRP)
Open/Closed Principle (OCP)
Liskov Substitution Principle (LSP)
Interface Segregation Principle (ISP)
Dependency Inversion Principle (DIP)
These principles aim to reduce code complexity, improve flexibility, and foster a design that adapts to change.
1. Single Responsibility Principle (SRP)
Definition: A class should have one and only one reason to change. In other words, a class should only have one responsibility.
Why it Matters:
Promotes focused, modular code.
Reduces the impact of changes in one part of the system on others.
Example: Bad Design:A class that handles both file operations and logging:
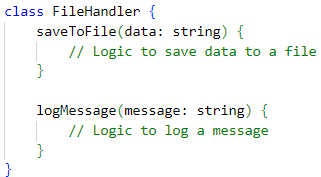
Good Design:Separate responsibilities into two classes:
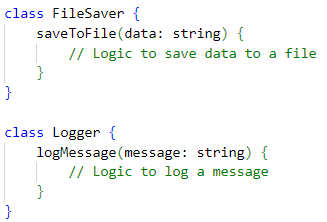
2. Open/Closed Principle (OCP)
Definition: A class should be open for extension but closed for modification. You should be able to add new functionality without altering existing code.
Why it Matters:
Enhances scalability.
Prevents breaking existing functionality when adding new features.
Example: Bad Design:Adding a new shape requires modifying the AreaCalculator:
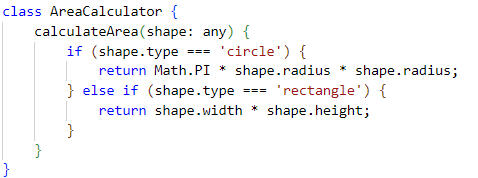
Good Design:Use polymorphism to extend functionality without modifying the calculator:
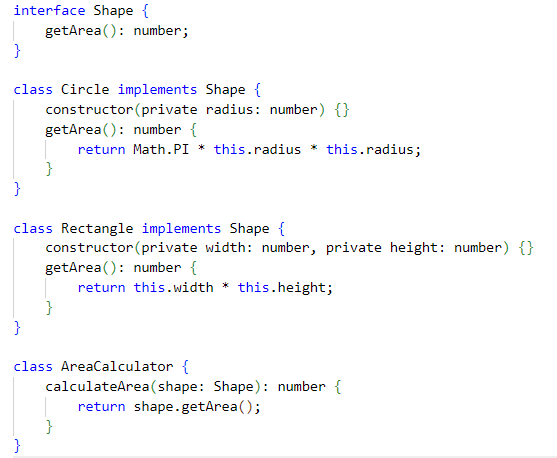
3. Liskov Substitution Principle (LSP)
Definition: Subtypes must be substitutable for their base types without altering the correctness of the program.
Why it Matters:
Ensures the program behaves consistently when using derived classes.
Prevents unexpected runtime errors.
Example: Bad Design:A subclass violates the behaviour of the base class:
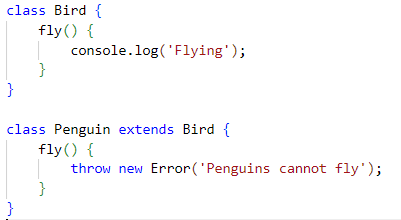
Good Design:Separate behaviours using interfaces:
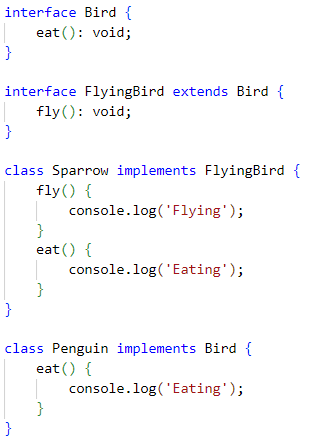
4. Interface Segregation Principle (ISP)
Definition: A class should not be forced to implement interfaces it does not use. Instead, create smaller, specific interfaces.
Why it Matters:
Prevents implementing unnecessary methods.
Simplifies code and enhances flexibility.
Example: Bad Design:A single interface forces classes to implement unrelated methods:
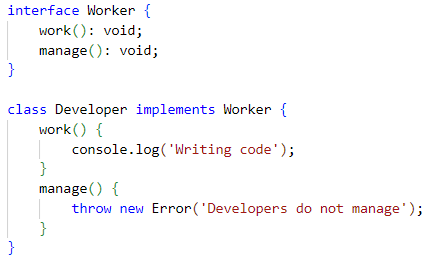
Good Design:Split the interface into focused contracts:
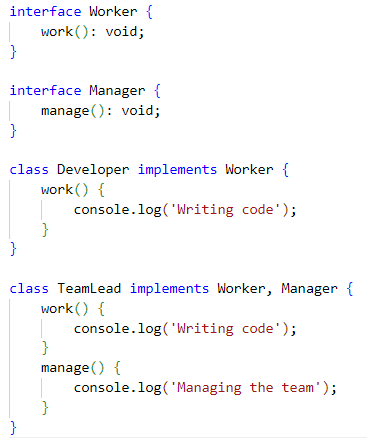
5. Dependency Inversion Principle (DIP)
Definition: High-level modules should not depend on low-level modules; both should depend on abstractions. Abstractions should not depend on details, but details should depend on abstractions.
Why it Matters:
Decouples modules, making them easier to test and modify.
Promotes reusable and scalable code.
Example: Bad Design:The high-level class depends directly on a low-level implementation:
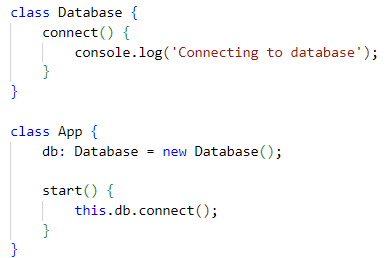
Good Design:Introduce abstractions to decouple the high-level module from low-level implementation:

Benefits of SOLID Principles
Improved Maintainability: Clean, modular code is easier to understand and modify.
Enhanced Scalability: Easily add new features without breaking existing functionality.
Increased Testability: Decoupled code allows isolated unit testing.
Better Collaboration: Simplified, focused classes make teamwork smoother.
When to Use SOLID Principles
Large Applications: Helps manage complexity in systems with multiple modules.
Evolving Projects: Accommodates frequent feature additions or changes.
Collaborative Development: Ensures uniformity and clear boundaries across teams.
Real-Life Applications of SOLID
E-Commerce Platforms: Managing product catalogs, orders, and payment systems with SRP and OCP.
Banking Systems: Implementing secure and extendable transaction modules using DIP.
Healthcare Software: Using ISP for modular, patient-focused systems.
Game Development: Applying LSP for game characters with varied behaviors.
Conclusion
SOLID principles are more than just theoretical guidelines; they are the cornerstone of effective software design. By incorporating these principles, developers can create systems that are not only robust but also adaptable to change, ensuring long-term success.
Whether you're building a startup application or a large-scale enterprise solution, SOLID principles help you write code that stands the test of time. Start applying these today and see the transformative impact on your projects.
Comments